Transform your Google Chrome homepage into a stunning and personalized landing page with quotes, wishes, and random background images with this article.
Pre-requisites:
-
Any code editor, preferably vscode
- Basics of HTML, CSS, and javascript
Here is a step-by-step procedure for building a simple landing page for any browser that makes you feel like you have a unique work style.
Prepare HTML document :
Firstly plan your landing page format of how you wish to have, then prepare an HTML document accordingly. Here is my HTML document for this project.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Hey Buddy</title>
<link rel="stylesheet" href="./style.css" />
</head>
<body>
<div class="clock-container">
<div class="time"></div>
<div class="date"></div>
<q id="quote"></q>
<p id="wish"></p>
</div>
<script src="script.js"></script>
</body>
</html>
Add styling to your project:
Style your HTML page as planned, here is my styling part to my web page.
@import url("https://fonts.googleapis.com/css?family=Heebo:300&display=swap");
* {
box-sizing: border-box;
}
html {
transition: all 0.5s ease-in;
}
body {
background: linear-gradient(rgba(0, 0, 0, 0.4), rgba(0, 0, 0, 0.4)),
url("https://source.unsplash.com/1600x900/?nature");
height: 100%;
background-repeat: no-repeat;
background-size: cover;
text-align: center;
display: flex;
justify-content: center;
align-items: center;
text-align: center;
color: #fff;
overflow: hidden;
}
.clock-container {
text-align: center;
width: 50rem;
max-width: 100%;
padding-top: 15%;
padding-bottom: 20%;
overflow: hidden;
justify-content: center;
align-items: center;
}
.time {
font-size: 7rem;
}
.date {
font-size: 3rem;
padding: 1rem;
}
#quote {
font-size: 1.8rem;
}
Follow my GitHub account for more Javascript projects https://github.com/kosarajunavya
Javascript functionality:
My Javascript code for this project is
const hourEl = document.querySelector(".hour"),
minuteEl = document.querySelector(".minute"),
secondEl = document.querySelector(".second"),
timeEl = document.querySelector(".time"),
dateEl = document.querySelector(".date"),
wish = document.getElementById("wish"),
toggle = document.querySelector(".toggle"),
days = ["Sunday","Monday","Tuesday","Wednesday","Thursday",
"Friday","Saturday"],
months = ["Jan","Feb","Mar","Apr","May","Jun","Jul","Aug","Sep",
"Oct","Nov","Dec"];
function setTime() {
const time = new Date(),
year = time.getFullYear(),
month = time.getMonth(),
day = time.getDay(),
date = time.getDate(),
hours = time.getHours(),
hoursForClock = hours >= 13 ? hours % 12 : hours,
minutes = time.getMinutes(),
seconds = time.getSeconds(),
ampm = hours >= 12 ? "PM" : "AM";
var wishTime = ["Good Morning", "Good Afternoon", "Good Evening"];
if (hours < 12) {
wish.innerHTML = `${wishTime[0]} Your name,what's your main focus today`;
} else if (hours < 17) {
wish.innerHTML = `${wishTime[1]} Your name, what's your main focus today`;
} else {
wish.innerHTML = `${wishTime[2]} Your name, what's your main focus today`;
}
setInterval(() => {
wish.innerHTML = "";
}, 60 * 60 * 1000);
timeEl.innerHTML = `${hoursForClock}:${
minutes < 10 ? `0${minutes}` : minutes
} ${ampm}`;
dateEl.innerHTML = `${days[day]} ${months[month]} <span>${date}</span> ${year}`;
setTime();
setInterval(setTime, 1000);
const url = "https://api.quotable.io/random";
function generateQuote() {
fetch(URL)
.then(function (data) {
return data.json();
})
.then(function (data) {
document.getElementById("quote").innerHTML = data.content;
})
.catch(function (err) {
console.log(err);
});
}
setInterval(generateQuote(), 10000);
Here is my source code for this project https://github.com/kosarajunavya/kosarajunavya.github.io/tree/main/wallpaper
Analyzing results:
Now go to the browser and check whether this functionality is working or not.
Perfect, there we go! We got an amazing landing page for any type of browser. Now the main part is how to add this project as your browser landing page. Look down for the procedure.
Adding this web page as a browser landing page:
Before going to add this beautiful landing page to your browser, you should save your file in git pages so that your project can be visible globally.
To know how to add your project to GitHub connect me to MyCode.blog.
After adding your project to GitHub you can get one global URL. My URL for this project is https://kosarajunavya.github.io/wallpaper/index.html
For chrome:
Now open Google Chrome on your PC/laptop, go to settings,
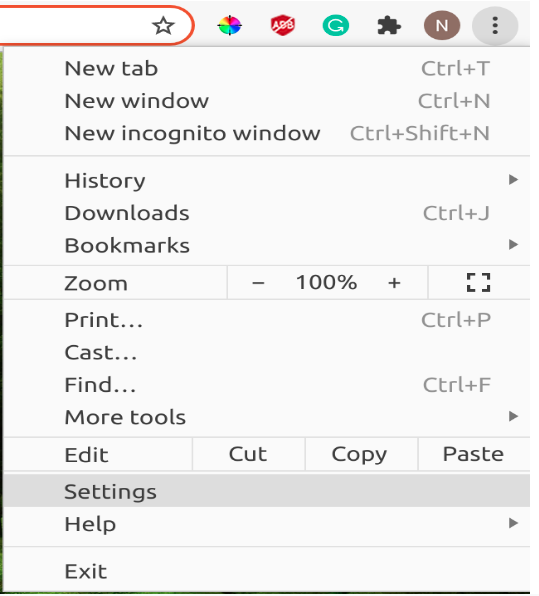
Select the on-start up option, you can see three options here, in that select Open a specific page or set of pages.
Next select Add a new page option, then you will be directed to the input form where you can place your GitHub URL.
Paste your GitHub URL here, that’s all. Now go open a new window in your chrome and you will be getting a nice landing page with some motivational quotations with a nice background and you will be getting a new quotation and background of every page reload.
Morning message:
Afternoon message:
Evening message:
For firefox:
In firefox go to settings,
Select home, and go to the Homepage and new windows then choose a custom URL.
Now you will be directed to place your project URL.
Then paste your URL and select use current page option, now open a new window in your browser and the magic.
Finally, I want to conclude that by following every single step without skipping, even a beginner can create a landing page for their PC/Laptop. So, go on guys, and get started.
Thank you so much for reading🤗! Hope you learned something from this article. Let me know if there's an issue or if I have missed something. I Will meet you in the comment section🙌!
FAQ
How does the quotation display a new one on every new open page or when the page refreshes?
In Javascript, we have an amazing concept called fetch. We can get any type of data from the external API, and with the help of getting methods, we will get the data from quotable.io random and display it on our landing page.
How does the background image update on every page refresh?
In Javascript, another cool method to get a new image on every page reload is getting Unsplash API and using it in the background image styling section.
Can I use this page as default for any type of browser?
Yes, you can use this page for any type of browser other than chrome and firefox. You can include some more features like adding a to-do list of your daily work or some major shortcuts.
How do the morning, afternoon, and evening messages get updated?
Since we are using the new Date() object, we can get the Indian Standard time, date, and current year. So based on the current running time value you can change the wish accordingly.
Comments