Learn how to validate the form inputs such as user name, password, email, and password confirmation along with a short note of the input status using JS and CSS.
While giving input to the form, sometimes without our knowledge we may miss a few inputs and that will result in the loss of certain data from the user. So we need to validate the form on submission. Here is the entire project of how we can validate the form on submission.
Recommended HTML structure
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="style.css" />
<title>Form Validator</title>
</head>
<body>
<div class="container">
<form id="form" class="form">
<h2>Register With Us</h2>
<div class="form-control">
<label for="username">Username</label>
<input type="text" id="username" placeholder="Enter username" />
<small>Error message</small>
</div>
<div class="form-control">
<label for="email">Email</label>
<input type="email" id="email" placeholder="Enter email" />
<small>Error message</small>
</div>
<div class="form-control">
<label for="password">Password</label>
<input type="password" id="password" placeholder="Enter password" />
<small>Error message</small>
</div>
<div class="form-control">
<label for="password2">Confirm Password</label>
<input
type="password"
id="password2"
placeholder="Enter password again"
/>
<small>Error message</small>
</div>
<button type="submit">Submit</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
In the above HTML structure, all the inputs are taken without any condition because we are validating the form using JS and there we are writing the conditions. We can also give the required conditions in the form input.
Required CSS for the project
style.css
:root {
--success-color: #2ecc71;
--error-color: #e74c3c;
}
* {
box-sizing: border-box;
}
body {
background-color: #f9fafb;
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
margin: 0;
}
.container {
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.3);
width: 400px;
}
h2 {
text-align: center;
margin: 0 0 20px;
}
.form {
padding: 30px 40px;
}
.form-control {
margin-bottom: 10px;
padding-bottom: 20px;
position: relative;
}
.form-control label {
color: #777;
display: block;
margin-bottom: 5px;
}
.form-control input {
border: 2px solid #f0f0f0;
border-radius: 4px;
display: block;
width: 100%;
padding: 10px;
font-size: 14px;
}
.form-control input:focus {
outline: 0;
border-color: #777;
}
.form-control.success input {
border-color: var(--success-color);
}
.form-control.error input {
border-color: var(--error-color);
}
.form-control small {
color: var(--error-color);
position: absolute;
bottom: 0;
left: 0;
visibility: hidden;
}
.form-control.error small {
visibility: visible;
}
.form button {
cursor: pointer;
background-color: #3498db;
border: 2px solid #3498db;
border-radius: 4px;
color: #fff;
display: block;
font-size: 16px;
padding: 10px;
margin-top: 20px;
width: 100%;
}
CSS is required for form validation, because if the user missed any input then we need to highlight the missed input along with some help text. Let us go for the actual functionality.
JS functionality
const form = document.getElementById("form");
const username = document.getElementById("username");
const email = document.getElementById("email");
const password = document.getElementById("password");
const password2 = document.getElementById("password2");
// Show input error message
function showError(input, message) {
const formControl = input.parentElement;
formControl.className = "form-control error";
const small = formControl.querySelector("small");
small.innerText = message;
}
// Show success outline
function showSuccess(input) {
const formControl = input.parentElement;
formControl.className = "form-control success";
}
// Check email is valid
function checkEmail(input) {
const re =
/^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
if (re.test(input.value.trim())) {
showSuccess(input);
} else {
showError(input, "Email is not valid");
}
}
// Check required fields
function checkRequired(inputArr) {
let isRequired = false;
inputArr.forEach(function (input) {
if (input.value.trim() === "") {
showError(input, `${getFieldName(input)} is required`);
isRequired = true;
} else {
showSuccess(input);
}
});
return isRequired;
}
// Check input length
function checkLength(input, min, max) {
if (input.value.length < min) {
showError(
input,
`${getFieldName(input)} must be at least ${min} characters`
);
} else if (input.value.length > max) {
showError(
input,
`${getFieldName(input)} must be less than ${max} characters`
);
} else {
showSuccess(input);
}
}
// Check passwords match
function checkPasswordsMatch(input1, input2) {
if (input1.value !== input2.value) {
showError(input2, "Passwords do not match");
}
}
// Get fieldname
function getFieldName(input) {
return input.id.charAt(0).toUpperCase() + input.id.slice(1);
}
// Event listeners
form.addEventListener("submit", function (e) {
e.preventDefault();
if (!checkRequired([username, email, password, password2])) {
checkLength(username, 3, 15);
checkLength(password, 6, 25);
checkEmail(email);
checkPasswordsMatch(password, password2);
}
});
In the above code, we are checking whether each and every input is provided or not on the form submission. if not provided we will be highlighting the input with the help text. In the same method, you can also apply the conditions for all other inputs if you need them.
Final Results:
Results of our project. The form is submitted with no inputs given results as shown in the below screenshot.
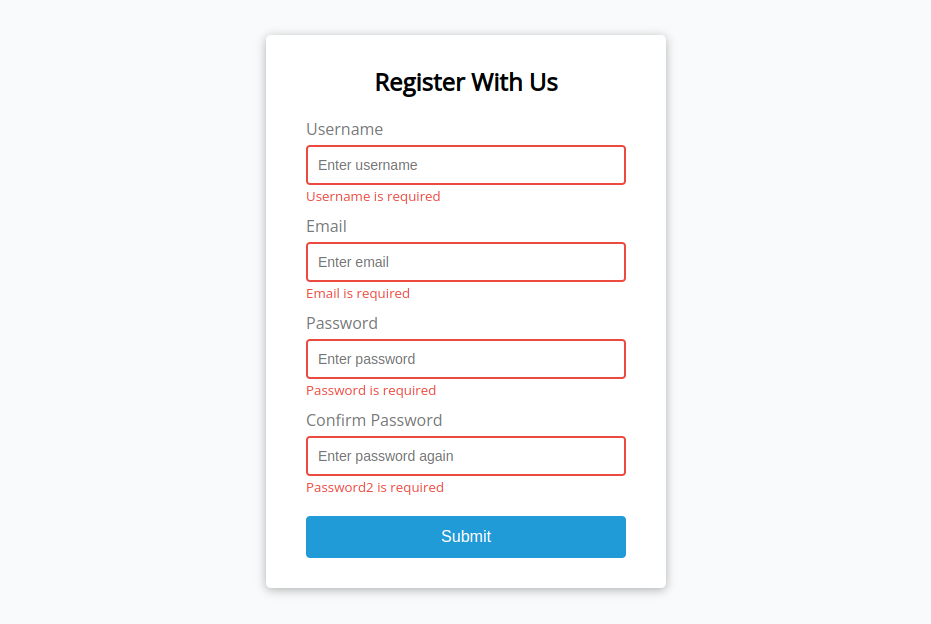
All inputs are given, and the passwords are also matched, but the given password strength has not matched the requirement. On form submission, password strength didn’t match will results as shown in the below screenshot.
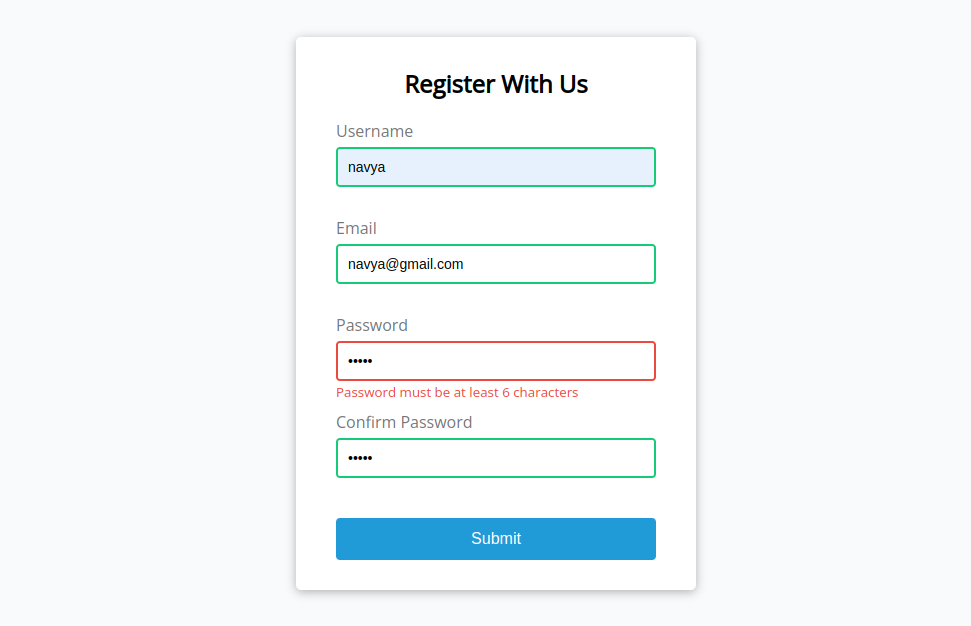
On form submission, if the passwords didn’t match will results as shown in the below screenshot.
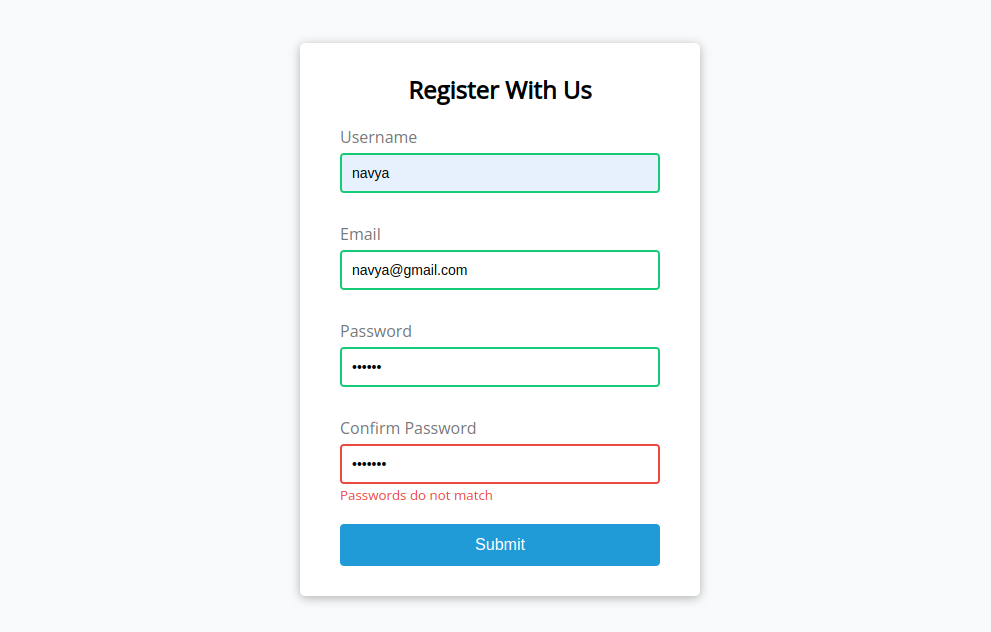
If the form is submitted without a proper email address, will results as shown in the below screenshot.
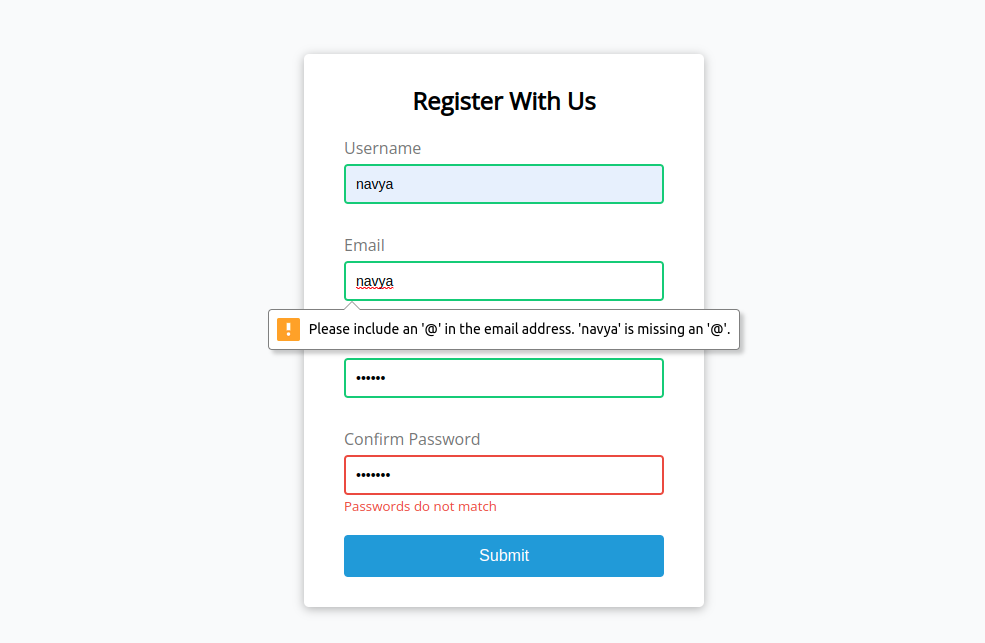
On form submission, if all the given inputs matched as per the requirement, will results as shown in the below screenshot.
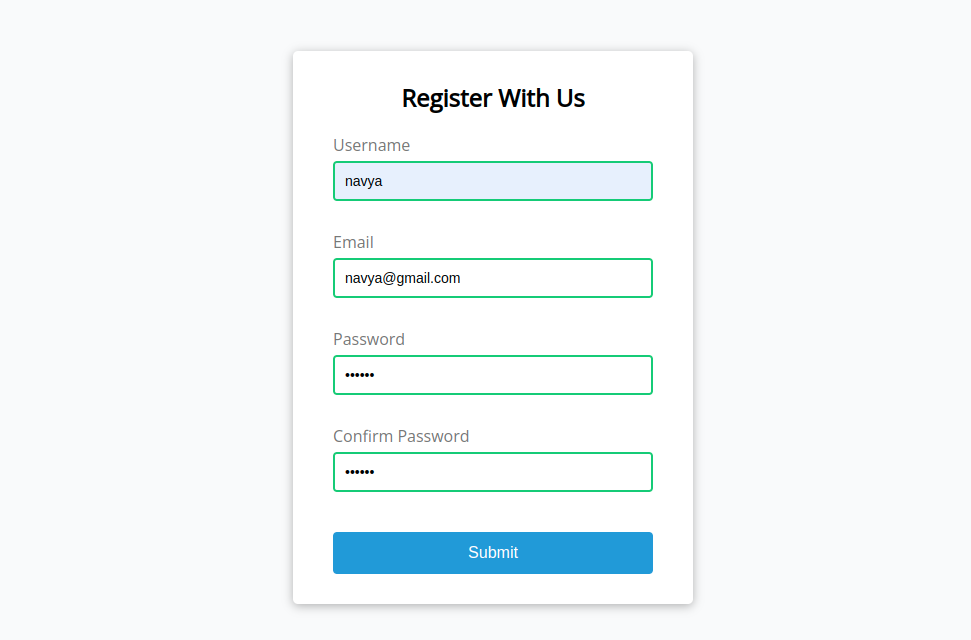
Finally, we have achieved the aim of the blog, which is validating the form based on the given inputs by the user. We have taken minimum conditions and applied for the inputs. You can also add the conditions for all the required inputs either using JS as given above or by using the required condition in the markup itself.
Comments