Building an admin form is a common first step in custom Drupal module development. It provides an adjustable settings interface for site editors/administrators.
This blog explains building an admin config form using a route, menu item, and saved form data in Drupal 8/9.
Create a custom module:
Modules in Drupal are located in the /modules folder. We'll create two custom and contrib folders inside the /modules folder. Additional modules from drupal.org will be stored in the contrib folder, and our custom modules will be stored in the custom folder.
Create a .info.yml file:
Let's name our custom module welcome_message. Create the folder /modules/custom/welcome_message. In this folder, we'll need to create the welcome_message.info.yml file and add the following code.
name: Welcome Message
description: Custom module for welcome Message.
type: module
core_version_requirement: ^8 || ^9
Enable a module:
For all possible ways to enable a module click here.
Using the Manage administrative menu, navigate to the Extend page in the list of modules, search the welcome page module and then select its checkbox. Scroll down to the bottom of the webpage, and then click Install.
Declaring a route:
The routing information is saved in welcome_message/welcome_message.routing.yml.
welcome_message.front_page:
path: '/welcome'
defaults:
_form: '\Drupal\welcome_message\Form\MessagesForm'
_title: 'WelcomeMessagesForm'
requirements:
_permission: 'access administration pages'
options:
_admin_route: TRUE
Let us take a closer look at what is contained in this YML file.
welcome_message.front_page: This is the route's machine name. Route machine names should be formatted as module_name.sub_name.
path: This is the URL of the page on your website.
defaults: This section describes the form and title callbacks.
requirements: This defines the conditions under which the form will be displayed.
Create an admin form:
Create the welcome_message\src\Form\MessagesForm.php file with the following code in it.
<?php
/**
* @file
* Contains Drupal\welcome_message\Form\MessagesForm.
*/
namespace Drupal\welcome_message\Form;
use Drupal\Core\Form\ConfigFormBase;
use Drupal\Core\Form\FormStateInterface;
class MessagesForm extends ConfigFormBase {
/**
* {@inheritdoc}
*/
protected function getEditableConfigNames() {
return [
'welcomemessage.adminsettings',
];
}
/**
* {@inheritdoc}
*/
public function getFormId() {
return 'welcome_form';
}
/**
* {@inheritdoc}
*/
public function buildForm(array $form, FormStateInterface $form_state) {
$config = $this->config('welcomemessage.adminsettings');
$form['welcome_message'] = [
'#type' => 'textarea',
'#title' => $this->t('Welcome message'),
'#description' => $this->t('Welcome message displays When a user login.'),
'#default_value' => $config->get('welcome_message'),
];
return parent::buildForm($form, $form_state);
}
/**
* {@inheritdoc}
*/
public function submitForm(array &$form, FormStateInterface $form_state) {
parent::submitForm($form, $form_state);
$this->config('welcomemessage.adminsettings')
->set('welcome_message', $form_state->getValue('welcome_message'))
->save();
}
}
Let us take a closer look at what is contained in this php file.
- ConfigFormBase is a base class for creating system configuration forms.
- getEditableConfigNames() is gets the configuration name.
- getFormId() is returns the form unique ID.
- buildForm() is returns the form array.
- validateForm() is validates the form.
- submitForm() is processes the form submission.
Add Form to menu system:
Menu items in Drupal 8/9 are defined in an a.yml file. To welcome_message.links.menu.yml, add the following:
welcome_message.admin_settings_form:
title: 'Welcome message configuration'
route_name: welcome_message.front_page
description: 'Welcome message admin'
parent: system.admin_config_system
weight: 99
Let us take a closer look at what is contained in this .menu.yml file.
- title: This will be displayed as a menu link.
- route_name: This route name is defined in welcome_message.routing.yml. This maps to the form class.
- description: It appears under the title.
- parent: This menu item will be nested beneath the parent menu item.
- weight: This determines the menu item's position in relation to its siblings.
Then clear the cache, you should see the form in the menu item under /admin/config/system.
Implement the form data:
For example, to display the saved welcome message to users when they login to the site. To accomplish this, you must include an implementation of hook_user_ login() to retrieve the configuration value. To obtain the module's configuration, call the \Drupal::config() static method with the module's configuration name (welcomemessage.adminsettings). Using the get() method, obtain the value of the welcome_message property.
Create the welcome_message.module under the welcome_message module and under the following code:
<?php
/**
* @file
* Contains welcome_message.module.
*/
/**
* Implement hook_user_login()
*/
function welcome_message_user_login() {
$config = \Drupal::config('welcomemessage.adminsettings');
\Drupal::messenger()->addMessage($config->get('welcome_message'));
}
Navigate to Configuration → system → Welcome Message Configuration.
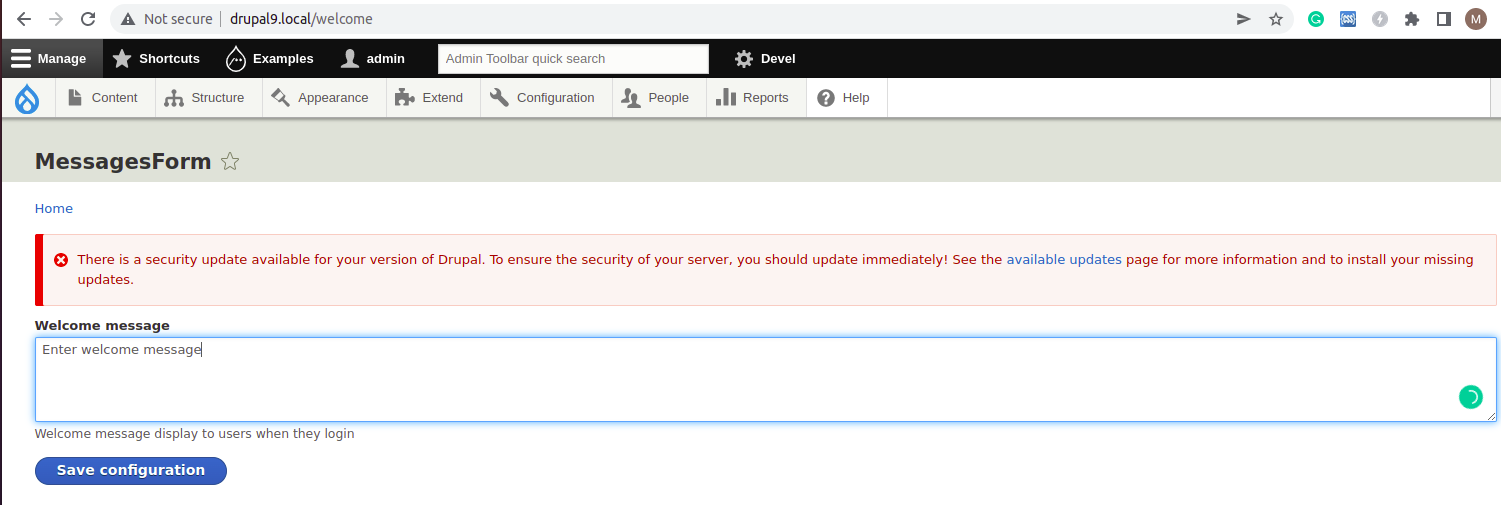
Enter the welcome message then click save configurations.
Next, clear the cache then log out of the site and log back in again, you should see the welcome message.
Conclusion:
That's all! You have successfully created an admin configuration form in Drupal 8/9.
Comments