Show/hide password functionality is a common feature on login pages, as it helps users to re-check their password and confirm while login, achieve it with jQuery.
Building the markup
First, we will construct the HTML structure with the required inputs needed for the login page. For example, I’m just going with two input fields only, that is username and password, and depending on the requirement you can add the input fields. Here is the structure of HTML.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>jQuery show/hide password functionality</title>
<!-- this is the jQuery cdn link -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
<link rel="stylesheet" href="./style.css" />
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.css"
integrity="sha512-5A8nwdMOWrSz20fDsjczgUidUBR8liPYU+WymTZP1lmY9G6Oc7HlZv156XqnsgNUzTyMefFTcsFH/tnJE/+xBg=="
crossorigin="anonymous"
/>
</head>
<body>
<div class="wrapper">
<form action="#" id="Login-form">
<h1>User Login Form</h1>
<div class="form-wrapper">
<div class="userName">
<label for="name">User Name</label>
<input
type="text"
pattern="[A-Za-z]{3}"
placeholder="userName / email"
/>
</div>
<div class="password">
<label for="password">Password</label>
<input type="password" id="password" class="form-control" /><i
class="toggle-hide-show fa fa-fw fa-eye-slash"
></i>
</div>
</div>
</form>
</div>
<script src="./script.js"></script>
</body>
</html>
When you are done with the html structure, just check for the results and analyze how it is reflected. Here is the screenshot for your reference.
It looks a bit ugly, with the plain HTML. So, let us add some colors to our login form to make it look good.
Basic styling
Before going to apply the actual functionality of show hide password in jquery, let us do some basic CSS to look pretty good compared to a plain one. Here is the basic CSS that I have applied for the project.
style.css
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
.wrapper {
display: flex;
justify-content: center;
align-items: center;
padding: 50px 0;
background: skyblue;
}
.userName {
padding: 30px;
}
.password {
padding: 0 30px 30px;
}
.wrapper form {
border: 1px solid #00000024;
background: #fff;
width: 600px;
max-width: 100%;
padding: 50px 0;
}
.wrapper h1 {
text-align: center;
}
.userName label,
.password label {
display: block;
padding-bottom: 10px;
}
.userName input,
.password input {
padding: 5px 10px;
}
.form-wrapper {
max-width: 50%;
display: block;
margin: 0 auto;
}
.toggle-hide-show {
position: relative;
right: 30px;
}
input:invalid {
border-color: red;
}
After applying all the above styles, let us check for the results, and here is the screenshot for your reference.
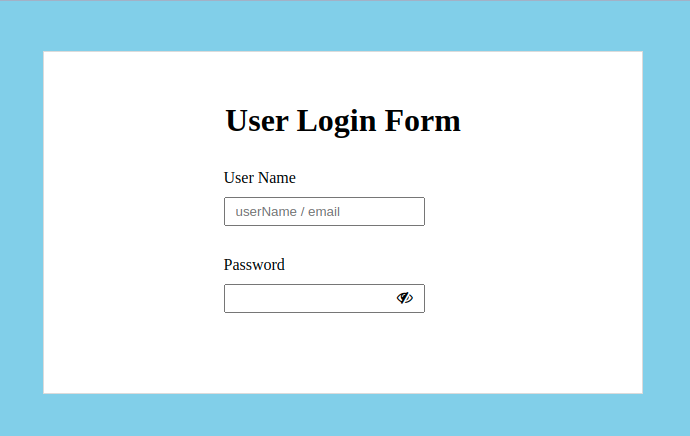
Wow, it looks better than the previous one. Now it’s time to apply for the show and hide password functionality.
jQuery functionality
Here is the required code for our project.
script.js
jQuery(".toggle-hide-show").click(function() {
jQuery(this).toggleClass("fa-eye fa-eye-slash");
input = jQuery(this).parent().find("input");
if (input.attr("type") == "password") {
input.attr("type", "text");
} else {
input.attr("type", "password");
}
});
Final results:
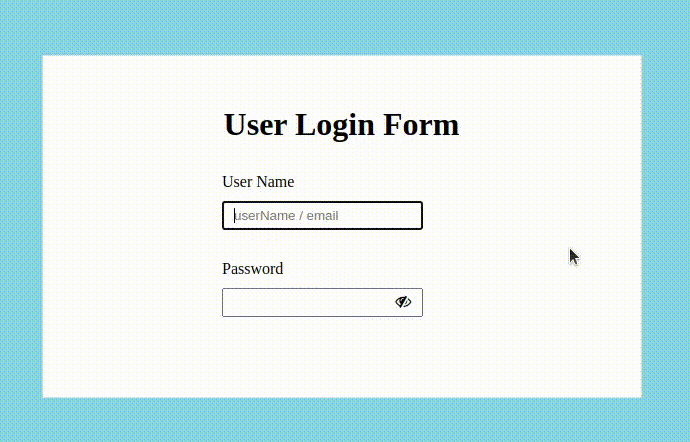
If you want to add autofocus to the first input of your form then click here to get the code.
Conclusion:
We have created a simple form with only two inputs, and applied the functionality of toggling showing and hiding the password. Hope you got the exact solution to your requirement, if you are stuck anywhere, please let us know our team will resolve your issues regarding the project.
Comments