Drupal 8/9 allows you to specify routes in a dynamic manner. To create dynamic routes, we initiate with the route_callbacks method in the routing.yml file.
Dynamic routes:
A method is used to implement dynamic routes. This method is added to module_name.routing.yml as a 'route_callbacks' entry.
route_callbacks:
- '\Drupal\module_name\Routing\MyModuleRoutes::routes'
Create src/Routing/MyModuleRoutes.php file:
The dynamic routing method either returns an array of \Symfony\Component\Routing\Route objects or a \Symfony\Component\Routing\RouteCollection object.
<?php
/**
* @file
* Contains \Drupal\module_name\Routing\MyModuleRoutes.
*/
namespace Drupal\module_name\Routing;
use Symfony\Component\Routing\Route;
/**
* Defines dynamic routes.
*/
class MyModuleRoutes {
/**
* {@inheritdoc}
*/
public function routes() {
$routes = [];
// Declares a single route under the name 'module_name.content'.
// Returns an array of Route objects.
$routes['module_name.content'] = new Route(
// Path to attach this route to:
'/hello_world',
// Route defaults:
[
'_controller' => '\Drupal\module_name\Controller\ExampleController::content',
'_title' => 'Hello'
],
// Route requirements:
[
'_permission' => 'access content',
]
);
return $routes;
}
}
Create src/Controller/ExampleController.php file:
As you can see, this simply adds another layer between routing.yml and the Controller. Our Controller appears to be as follows:
<?php
namespace Drupal\module_name\Controller;
use Drupal\Core\Controller\ControllerBase;
class ExampleController extends ControllerBase {
/**
* @return string[]
*/
public function content() {
return [
'#markup' => 'Hello world ?',
];
}
}
Finally, we goto URL and navigate to /hello_world page then the output looks as follows:
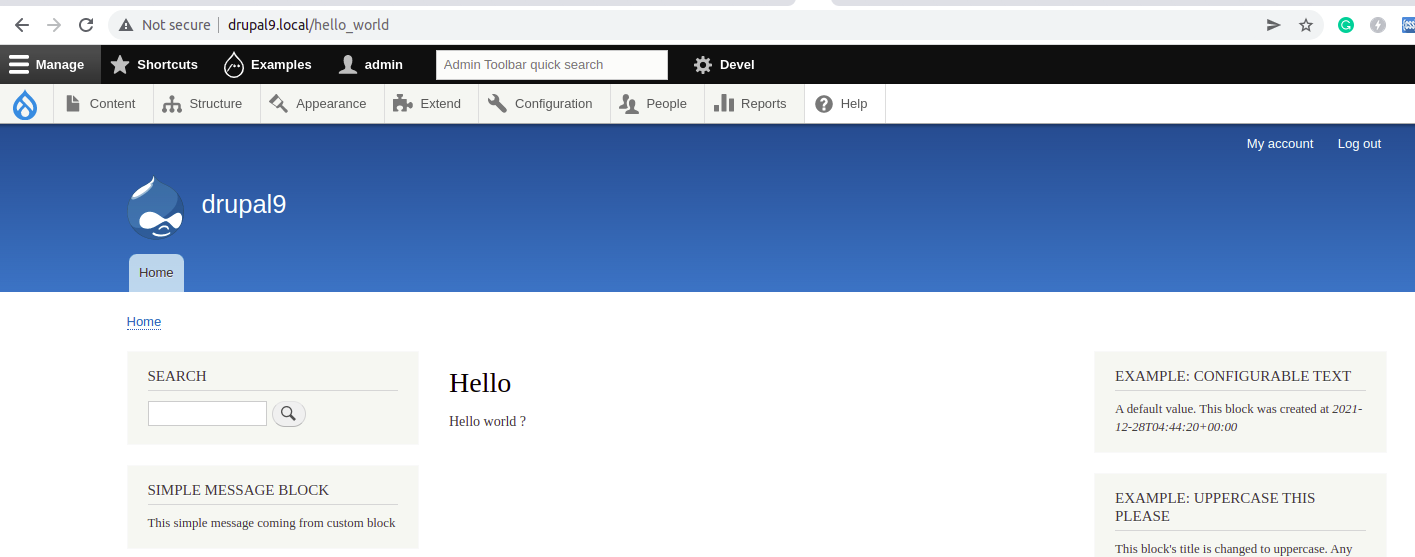
Conclusion:
In Drupal 8/9 create dynamic routes by initiating with the route_callbacks method in the routing.yml file.
Comments